Using Microsoft Azure Entity Linking Cognitive API
Microsoft Cognitive Services
Microsoft Cognitive Services, formerly known as Project Oxford
are a set of APIs, SDKs and services available to developers to make their applications more intelligent, engaging and discoverable. These services let you build apps with powerful algorithms to see, hear, speak, understand and interpret using natural methods of communication, with just a few lines of code. Microsoft Cognitive Services enable the developers to easily add intelligent features such as:
- Emotion and video detection
- Facial, speech and vision recognition
- Speech and language understanding
Microsoft Entity Linking Intelligence Service API
This API is part of the broader set of APIs provided as part of Microsoft Cognitive Services
APIs. This API is designed to help developers with tasks related to Entity Linking
. One of the challenges while working with Natural Language Processing is recognizing the context of a particular word within a paragraph/sentence, a word might be used differently in different contexts. For example, in the case where “times” is a named entity, it still may refer to two separately distinguishable entities, such as “The New York Times” or “Times Square”. Given a specific paragraph the Entity Linking Intelligence Service
will recognize and identify each separate entity based on its context.
Install Microsoft.ProjectOxford.EntityLinking Package
Make sure you have added the Microsoft.ProjectOxford.EntityLinking
NuGet Package to your project.
To add the pacakge Go to Tools
> Nuget Package Manager
> Package Manager Console
Install-Package Microsoft.ProjectOxford.EntityLinking
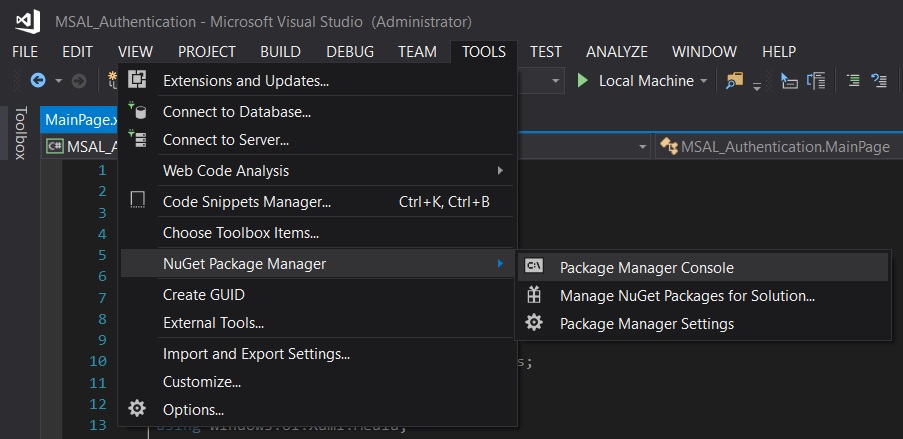
Get an API Subscription Key
A subscription to Entity Linking Intelligence Service API is required to use the service. A sign up may be required if don’t already have an account. To get a subscription key you can visit the link below and follow the steps below.
- Goto Try Cognitive Services
- Click
Get API Key
, Read and Agree to Microsoft Cognitive Services Terms. - Sign-in using your preferred account.
- Once Signed-in you will be redirected to a web page as seen below in the screenshot below.
- Save this information and either of the keys can be used to call the API Service.
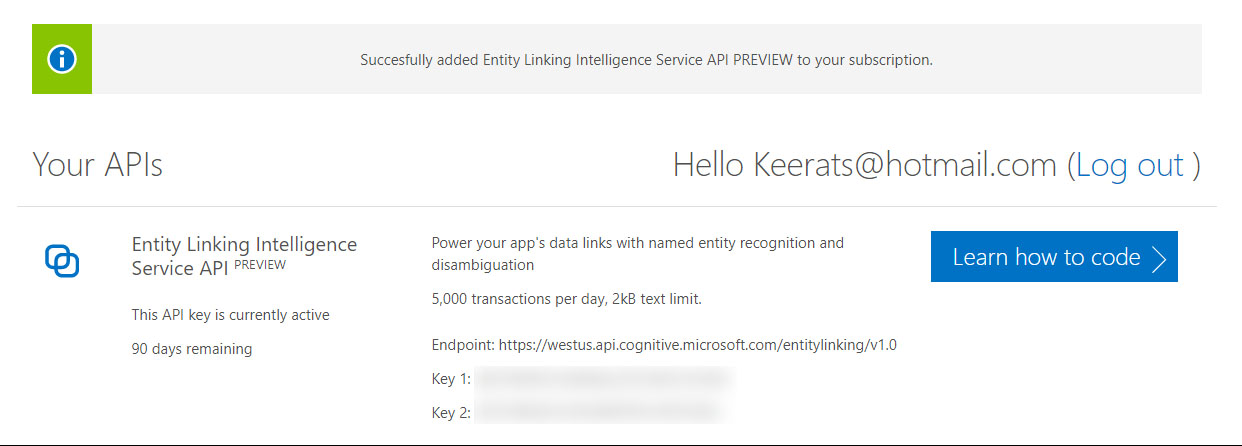
Creating an EntityLinkingServiceClient object
The very first step is to create an object of the class EntityLinkingServiceClient
, there are various overloaded constructors that can be used to instantiate the object, however, we will use the version that takes only one argument as input, i.e. an API Subscription Key
.
This is the same subscription key that was generated in Step 1.
public static EntityLinkingServiceClient clientApplication;
clientApplication = new EntityLinkingServiceClient("Enter your Subscription Key here");
Calling the Microsoft Azure Entity Linking Cognitive API
Once we have the service client initialized, the next step is to call the LinkAsync
method. Along with the text, you want to identify entities from, you can also specify a specific word within that text that you want to be entity linked. However, if you specify a specific word, you will also have to specify the location of the specific word in the given text.
This method takes the following arguments:
public Task<EntityLink[]> LinkAsync(string text, string selection = "", int offset = 0);
- string text // Text that is supplied to the service to separate the entities from.
- string selection // Specific word within the text you want to be entity linked
- int offset // position of the specific word offset by the number of character within the given text.
In a case where only one argument, the input text is specified, then the service tries to recognize and identify all the entities as seen in the code below.
var linkResponse = await App.clientApplication.LinkAsync(txtRequest.Text);
Display Entity Linking API Response
The response from the method LinkAsync
call is returned in the form of an array of objects of the type EntityLink
.
The objects in the array are traversed one by one, serialized and formatted into JSON format.
foreach (var entity in linkResponse)
{
// Serialize and format the response into JSON.
resultJSON.Append(JsonConvert.SerializeObject(entity) + "\n");
}
We also use a LINQ
query to retrieve the WikipediaID from each Entity, these IDs are then displayed accordingly.
// Retrieve Wikipedia IDs for all the Entities.
var resultEnitites = string.Join("\n", linkResponse.Select(i => i.WikipediaID).ToList());
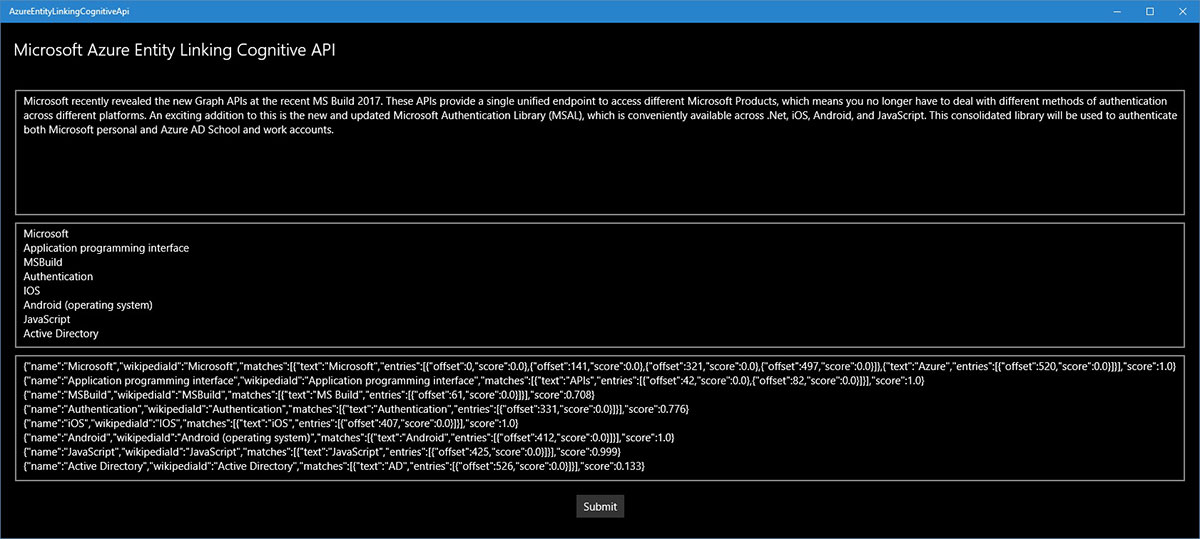
Source Code
You can find the source code at Github AzureEntityLinkingCognitiveApi repository as Visual Studio 2017
solution.
Final note
This is a very brief introduction to get you started using the Microsoft Azure Entity Linking Cognitive API. The same method can be improvised upon and used to connect to other Cognitive APIs. If you have any questions or concerns, feel free to leave a comment.